Project: Cracking Password Vaults
Objectives
You have managed to breach the storage of a password manager company and obtained encrypted vaults and password hashes for each of their users. You have some particular vaults that you are interested in decrypting because they contain the username and password for some accounts for “interesting” individuals. You believe these individuals may have a weak password that may appear in a separate list of breached passwords you have acquired.
Project Setup
Download the handout for this assignment. This contains:
- a folder called
practice
, which contains a practice vault and the password for this vault - a folder called
breach
, which contains:- a set of vaults that you have obtained from a password manager company
- a set of passwords that you have separately obtained from a password breach dataset
Password Vaults
The password vaults have been encrypted with the following process:
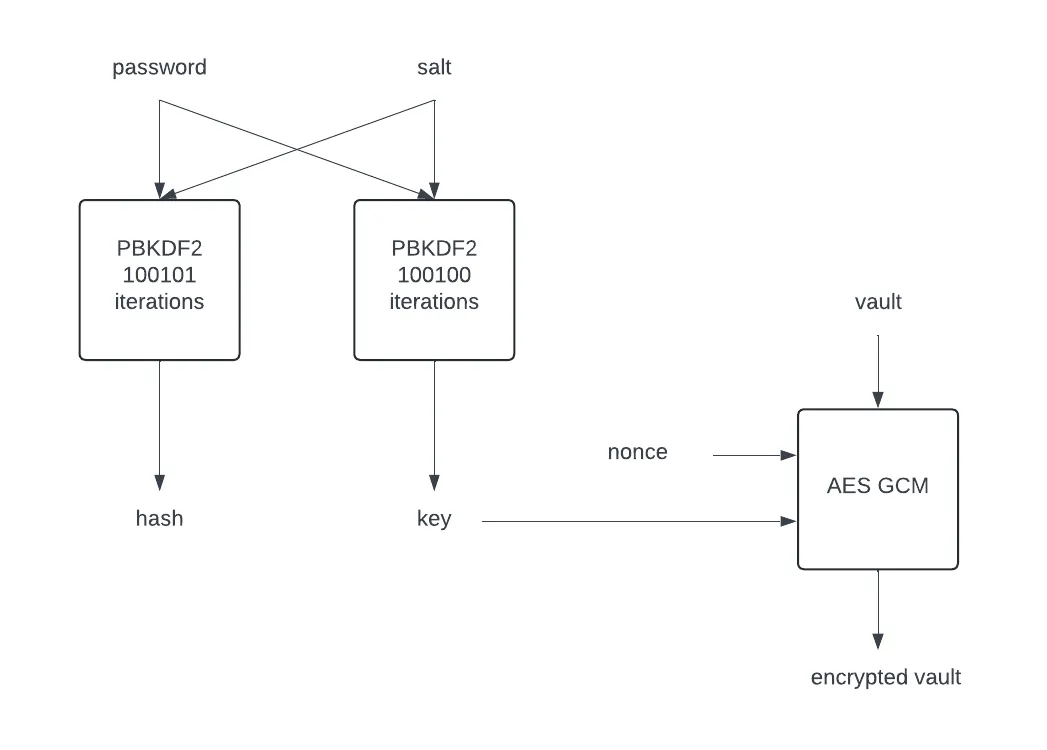
- the password and a random salt are used to derive a hash and a key with
PBKDF2, a password-based key derivation function
- the system uses 100,100 iterations of PBKDF2 to derive a key
- the system uses 100,101 iterations of PBKDF2 to derive a hash
- the key and a random nonce are used to encrypt the vault using AES GCM
The salt, hash, nonce, and vault are coded into hex values.
The password manager company then stores password vaults and their associated information in this format:
username:hex_salt:hex_hash:hex_nonce:hex_vault
Practice Vault
As a first step, write code to decrypt the practice vault. You have the password for this vault, so you can work through the steps to decrypt a vault:
-
convert the salt, nonce, and vault from hex to byte arrays using the base16ct crate
- the salt is 12 bytes of
u8
- the nonce is 12 bytes of
u8
- the vault is variable size, so convert it to a vector of
u8
- the salt is 12 bytes of
-
use the salt and the password with pbkdf2 to generate the key and the hash
-
convert the hash to hex and compare it to the hash from the vault to be sure they match
-
use the key and the nonce with AES GCM to decrypt the vault
-
print the username and the vault contents
Breach Vaults
For each of of the vaults, try each of the breach passwords to see if one of them works to decrypt the vault. Note: - if the hash doesn’t match, this is not the right password - if the hash does match, the vault should be able to be decrypted with the nonce and key, so you don’t need to try any more passwords. It is possible none of the passwords match for any given vault.
You should be able to crack 3 of the 6 vaults.
Grading Rubric
- Total (40 points)
- 10 points for each vault you can crack
Submission
- Submit the output of your cracking program, which should show us the contents of all of the cracked vaults. It is OK if you also show every password as you check it for each vault.
- Submit your code.
Use the tar
command and your BYU netID to compress your files:
tar --exclude=target --exclude=.git -czvf byuNetID.tar directory
Submit your tar file on Learning Suite.